How to Automatically Create UML Class Diagrams from Requirements Using ChatGPT
You probably know that ChatGPT can write code. But did you know it can create class diagrams too?
This is possible using PlantUML syntax. PlantUML is a software engineering tool used to create UML diagrams, including class diagrams. It uses a simple text syntax to describe the diagram elements, which are then rendered into graphical representations.
PlantUML is a convenient tool even without auto-generation. You can check examples at https://www.planttext.com to see how it works in practice.
Object Oriented Analysis and Design using ChatGPT
Object-Oriented Analysis and Design is a method used in software engineering to design and develop software systems. It emphasizes the use of objects, classes, and their relationships to model the system and its behavior.
UML Class diagrams are a key component of the software design. They are used to modeling the structure of a system by defining the classes, their attributes and methods, and the relationships between them. Class diagrams provide a graphical representation of the system’s structure. They make it easier to understand and communicate about the system.
Creating a class diagram involves identifying the classes, attributes, methods, and relationships that make up the system and then organizing them into a diagram.
I don’t recommend trying to design the entire system upfront. Instead, it’s better to iterate and improve the design while coding. However, I still recommend doing some rough planning before writing code. This will make your thoughts clearer and give you an idea of what the system’s design will look like.
To identify the classes and to create a class diagram, you need to have requirements of the system available in some form.
ChatGPT is already quite good at identifying classes and their relationships from textual input. Let’s see how this work in practice.
UML class diagram creation from textual input
In this example, we create a class diagram for a movie database app using ChatGPT. The app is for creating movie watchlists and showing helpful stats from those movies.
The app is designed for exercise, and its requirements are publicly available at https://github.com/florinpop17/app-ideas/blob/master/Projects/3-Advanced/Movie-App.md.
In this example, I have copy-pasted the original requirements for ChatGPT without editing them first.
Prompt:
Create a class diagram for the following requirements using PlantUML
syntax. Show all the relationships between the classes. For instance,
if there is a property List<User>, display the aggregation between
the User class and the class containing the property. Present the
output as a code block.
Requirements:
"""
Movie Database App
Find your next movie or create your watchlist with this App.
It include reviews, rating, actors and anything you need to know
about the movie.
This application will help users find their next movie to watch by
showing helpful stats. Resource needed for the project is movie api,
examples include Imdb, MovieDB etc.
User Stories
User can see all the latest movie on the front page
User scroll down to see all other movies according to release date
User can click on any of the movie to go to their own separate page
User can then see all about the movie ratings, about, actors
present on each separate movie page
Bonus features
User can create an account
User can create their own watch list
User can review movies
Useful links and resources
MovieDB Api
"""
Output:
@startuml
class User {
+username: String
+password: String
+createAccount()
+createWatchList()
+reviewMovie()
}
class Movie {
+id: String
+title: String
+releaseDate: Date
+rating: Float
+about: String
+actors: List<Actor>
+getDetails()
}
class Actor {
+name: String
+getDetails()
}
class Review {
+userId: String
+movieId: String
+rating: Float
+comment: String
+submitReview()
}
class WatchList {
+userId: String
+movies: List<Movie>
+addMovie()
+removeMovie()
}
class MovieAPI {
+sourceName: String
+getLatestMovies()
+getMovieDetails()
}
User "1" -- "0..*" Review : writes >
User "1" -- "1" WatchList : owns >
Review "0..*" -- "1" Movie : reviews >
WatchList "1" -- "0..*" Movie : contains >
Movie "1" -- "0..*" Actor : has >
MovieAPI ..> Movie : provides >
@enduml
You can copy and paste the output to planttext.com to visualize the result quickly.
Graphical presentation:
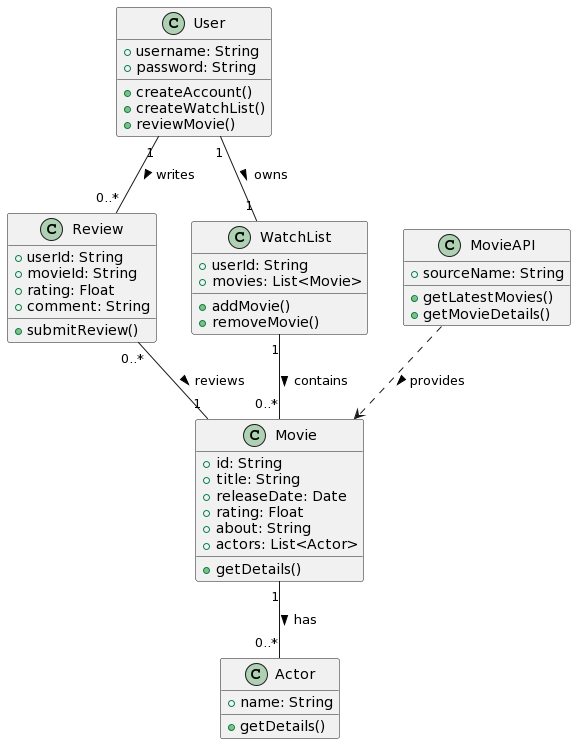
The class diagram of the Movie Database App generated by ChatGPT
As you can see, the result is quite good. It’s not perfect. For example, the MovieAPI provides only two actions. But in just a few minutes, you can get an idea of what the class structure of the app could look like. And by running the prompt multiple times, you can get alternative versions to make choice from.
This is a great way to get started quickly. You’ll see what the design of your app could look like if you already have requirements or user stories in textual format.
From here, you can continue by manually refining the class diagram or by instructing ChatGPT to edit the diagram in a way you want.
Bonus tip 1: Generate Python code from the class diagram
When you have a class diagram, you can also quickly generate initial code using ChatGPT. Here’s an example of how to do it.
Prompt:
Based on the class diagram, write a backend implementation
using Python and Flask
Output:
Sure, here is a very basic implementation of the classes using Python and Flask. Note that this is a simplified example and a real-world implementation would involve more complex code with error handling, security features, and database interaction.
app.py
:
from flask import Flask, request
from flask_sqlalchemy import SQLAlchemy
from sqlalchemy.orm import relationship
app = Flask(__name__)
app.config['SQLALCHEMY_DATABASE_URI'] = 'sqlite:////tmp/test.db'
db = SQLAlchemy(app)
class User(db.Model):
id = db.Column(db.Integer, primary_key=True)
username = db.Column(db.String(80), unique=True, nullable=False)
password = db.Column(db.String(120), nullable=False)
reviews = relationship("Review", backref="user")
watchlist = relationship("WatchList", uselist=False, back_populates="user")
class Movie(db.Model):
id = db.Column(db.Integer, primary_key=True)
title = db.Column(db.String(120), nullable=False)
release_date = db.Column(db.DateTime, nullable=False)
rating = db.Column(db.Float, nullable=False)
about = db.Column(db.String(500), nullable=False)
actors = db.Column(db.String(500), nullable=False)
reviews = relationship("Review", backref="movie")
watchlists = relationship("WatchList", back_populates="movies")
class Review(db.Model):
id = db.Column(db.Integer, primary_key=True)
user_id = db.Column(db.Integer, db.ForeignKey('user.id'))
movie_id = db.Column(db.Integer, db.ForeignKey('movie.id'))
rating = db.Column(db.Float, nullable=False)
comment = db.Column(db.String(500), nullable=True)
class WatchList(db.Model):
id = db.Column(db.Integer, primary_key=True)
user_id = db.Column(db.Integer, db.ForeignKey('user.id'))
movie_id = db.Column(db.Integer, db.ForeignKey('movie.id'))
user = relationship("User", back_populates="watchlist")
movies = relationship("Movie", back_populates="watchlists")
@app.route('/user', methods=['POST'])
def create_user():
data = request.get_json()
new_user = User(username=data['username'], password=data['password'])
db.session.add(new_user)
db.session.commit()
return {"message": "User created successfully."}, 201
@app.route('/movie', methods=['POST'])
def add_movie():
data = request.get_json()
new_movie = Movie(title=data['title'], release_date=data['release_date'], rating=data['rating'], about=data['about'], actors=data['actors'])
db.session.add(new_movie)
db.session.commit()
return {"message": "Movie added successfully."}, 201
@app.route('/review', methods=['POST'])
def add_review():
data = request.get_json()
new_review = Review(user_id=data['user_id'], movie_id=data['movie_id'], rating=data['rating'], comment=data['comment'])
db.session.add(new_review)
db.session.commit()
return {"message": "Review added successfully."}, 201
@app.route('/watchlist', methods=['POST'])
def create_watchlist():
data = request.get_json()
new_watchlist = WatchList(user_id=data['user_id'], movie_id=data['movie_id'])
db.session.add(new_watchlist)
db.session.commit()
return {"message": "Watchlist created successfully."}, 201
if __name__ == "__main__":
db.create_all()
app.run(debug=True)
Please note, that for simplicity, this example uses SQLite as the database and doesn’t include fetching data from any external Movie API. Also, this code does not include any form of authentication or validation, which you would want to add in a real-world application.
Again, the result is not a ready application that you can just deploy. We can also see that is not exactly 100% based on the class diagram. But for prototypes, it’s a quick way to get started.
Bonus tip 2: Auto-Generate class diagram from Python code
If you already have code that you would like to generate a class diagram from, you can do that as well. Just copy and paste the code of each file separately to the input. Please note that the maximum input size limit restricts auto-generation of class diagrams from large code bases.
Prompt:
Create a class diagram for the following code using PlantUML
syntax. Show all the relationships between the classes.
Code:
"""
<paste your code here>
"""
As a result, ChatGPT will output a class diagram that is very similar to the original. You can try to see it yourself.
Conclusion
ChatGPT can generate class diagrams and initial code quickly. This can be helpful if you want to get ideas about what the design could look like or prototype an application quickly.
The result still needs some manual work to finish, but ChatGPT can save you a huge amount of time. I recommend you to try it. By experiencing it yourself, you’ll notice how it best suits your purposes and find new ways to enhance your work.
Explore More
- Class Diagrams (Wikipedia)
- ChatGPT
- PlantUML
- PlantText